Java Objects & Classes :
Java is an Object-Oriented Language. As a language that has the Object-Oriented feature, Java supports the following fundamental concepts −
- Polymorphism
- Inheritance
- Encapsulation
- Abstraction
- Classes
- Objects
- Instance
- Method
- Message Passing
Objects in JAVA :
Everything in Java is associated with classes and objects, along with its attributes and methods. For example: in real life, a car is an object. The car has attributes, such as weight and color, and methods, such as drive and brake. A Class is like an object constructor, or a “blueprint” for creating objects.
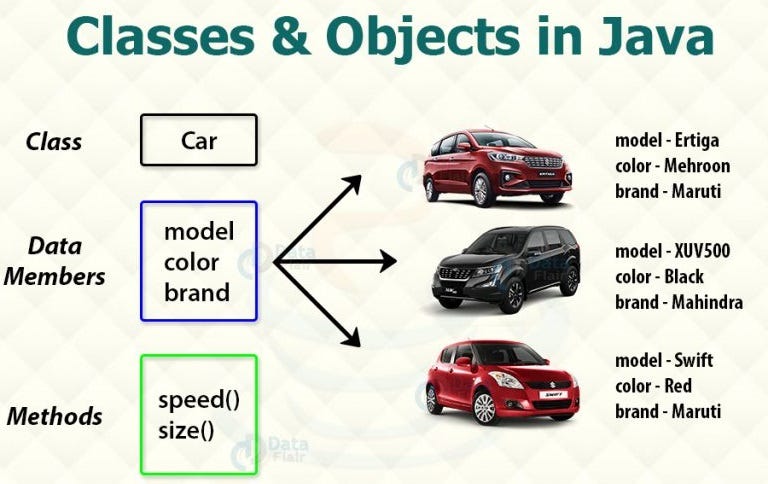
Classes in Java
A class is a blueprint from which individual objects are created.
public class Dog {
String breed;
int age;
String color;
void barking() { …. }
void hungry() { …. }
void sleeping() { …. }
}
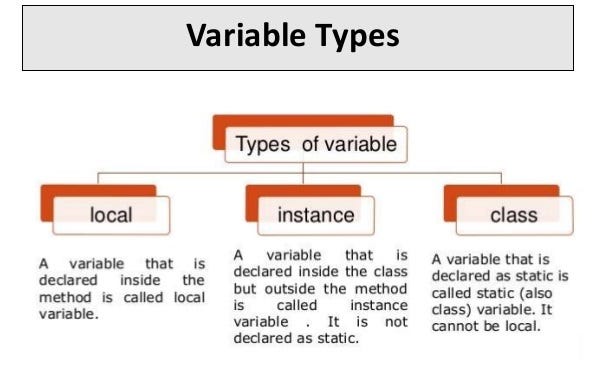
Constructors
One of the most important sub topic would be constructors. Every class has a constructor. If we do not explicitly write a constructor for a class, the Java compiler builds a default constructor for that class. A constructor is used to initialize the state of an object.
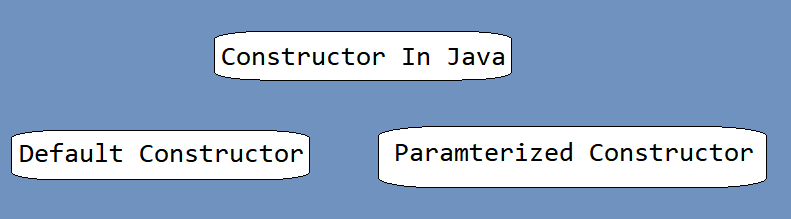
A class can have more than one constructor.
public class Dog{
public Dog() {
}
public Dog(String name) {
// This constructor has one parameter
}
}
Example :explaining how to access instance variables and methods of a class.
public class Puppy {
int puppyAge;
public Puppy(String name) {
// This constructor has one parameter, name.
System.out.println("Name chosen is :" + name );
}
public void setAge( int age ) {
puppyAge = age;
}
public int getAge( ) {
System.out.println("Puppy's age is :" + puppyAge );
return puppyAge;
}
public static void main(String []args) {
/* Object creation */
Puppy myPuppy = new Puppy( "tommy" );
/* Call class method to set puppy's age */
myPuppy.setAge( 2 );
/* Call another class method to get puppy's age */
myPuppy.getAge( );
/* You can access instance variable as follows as well */
System.out.println("Variable Value :" + myPuppy.puppyAge );
}
}
Case Study
For our case study, we will be creating two classes. They are Employee and EmployeeTest.
First open notepad and add the following code. Remember this is the Employee class and the class is a public class. Now, save this source file with the name Employee.java.
The Employee class has four instance variables — name, age, designation and salary. The class has one explicitly defined constructor, which takes a parameter.
Java Package
In simple words, it is a way of categorizing the classes and interfaces. When developing applications in Java, hundreds of classes and interfaces will be written, therefore categorizing these classes is a must as well as makes life much easier.
Import Statements
In Java if a fully qualified name, which includes the package and the class name is given, then the compiler can easily locate the source code or classes. Import statement is a way of giving the proper location for the compiler to find that particular class.
we are using java.io.package in next Example —
Example
import java.io.*;
public class Employee {
String name;
int age;
String designation;
double salary;
// This is the constructor of the class Employee
public Employee(String name) {
this.name = name;
}
// Assign the age of the Employee to the variable age.
public void empAge(int empAge) {
age = empAge;
}
/* Assign the designation to the variable designation.*/
public void empDesignation(String empDesig) {
designation = empDesig;
}
/* Assign the salary to the variable salary.*/
public void empSalary(double empSalary) {
salary = empSalary;
}
/* Print the Employee details */
public void printEmployee() {
System.out.println("Name:"+ name );
System.out.println("Age:" + age );
System.out.println("Designation:" + designation );
System.out.println("Salary:" + salary);
}
}
Employee class there should be a main method and objects should be created. We will be creating a separate class for these tasks.
import java.io.*;
public class EmployeeTest {
public static void main(String args[]) {
/* Create two objects using constructor */
Employee empOne = new Employee("James Smith");
Employee empTwo = new Employee("Mary Anne");
// Invoking methods for each object created
empOne.empAge(26);
empOne.empDesignation("Senior Software Engineer");
empOne.empSalary(1000);
empOne.printEmployee();
empTwo.empAge(21);
empTwo.empDesignation("Software Engineer");
empTwo.empSalary(500);
empTwo.printEmployee();
}
}
NOTE : Save files as “ Class name.java“ format
Output :
C:\> javac Employee.java
C:\> javac EmployeeTest.java
C:\> java EmployeeTest
Name:James Smith
Age:26
Designation:Senior Software Engineer
Salary:1000.0
Name:Mary Anne
Age:21
Designation:Software Engineer
Salary:500.0
For Reference ,
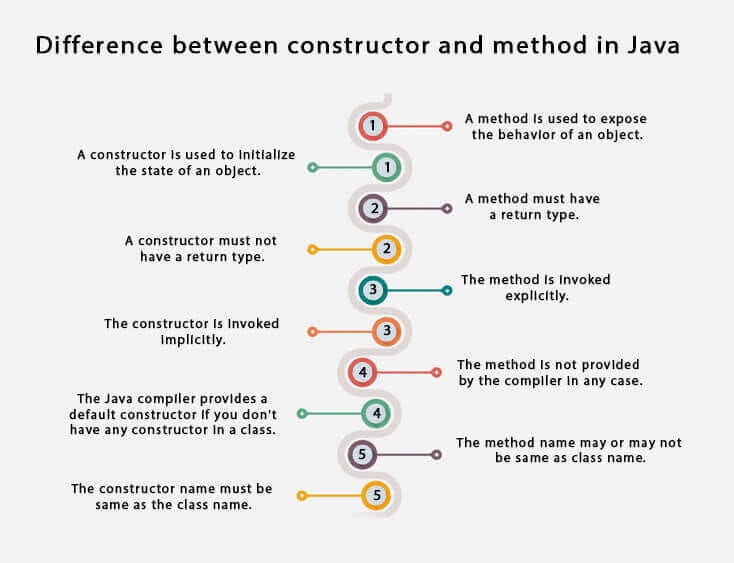
Check Out my YouTube channel for HackerRank Solutions In JAVA .
Good work.. keep it up
ReplyDeleteThanks ☺️
ReplyDeleteUseful Information
ReplyDeleteMast
ReplyDelete